- Today
- Total
프로그래밍 농장
유데미 스타터스 유니티 1기 취업 부트캠프 - 13주차 학습 일지 본문
이번주는 저번주에 이어 최종적으로 MagicaVoxel 오브젝트들을 이용한 프로젝트를 완성하였다.
2주가까이 다양한 Voxel 오브젝트들을 만들고 배우면서 쌓은 것들을 기반으로하여 프로젝트를 완성할수있어서 뿌듯했다 !
초반 게임 시작 및 로그인 Scene은 아래와 같으며, Resources.Load() 함수를 통하여, 프로젝트 내 폴더의 다양한 파일들을 불러와, 배경화면을 전환시켜주도록 구현하였다.
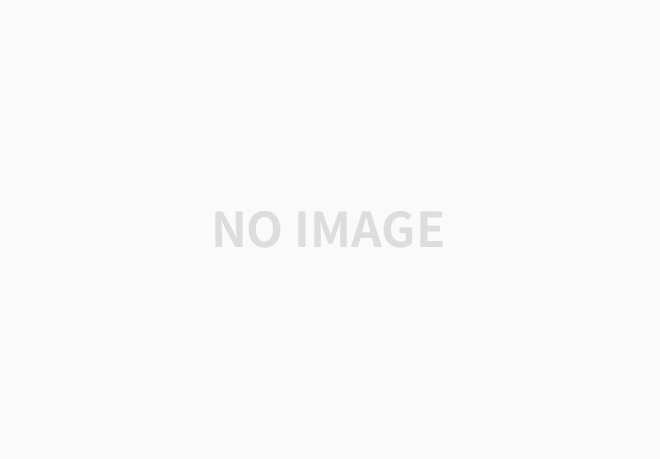
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class SpriteChange : MonoBehaviour
{
Image Wallpaper;
int cnt = 0;
public Sprite[] sprites;
// Start is called before the first frame update
void Start()
{
Wallpaper = GetComponent<Image>();
sprites = Resources.LoadAll<Sprite>("Sprite_wallpaper/");
InvokeRepeating("wallpaper_Change", 2f,2f);
}
// Update is called once per frame
void Update()
{
}
void wallpaper_Change()
{
if (cnt == 3)
{
cnt = 0;
Wallpaper.sprite = sprites[cnt];
cnt++;
}
else
{
Wallpaper.sprite = sprites[cnt];
cnt++;
}
}
}
로그인 후에는, 아래와 같이 플레이할 캐릭터를 선택할수있다.
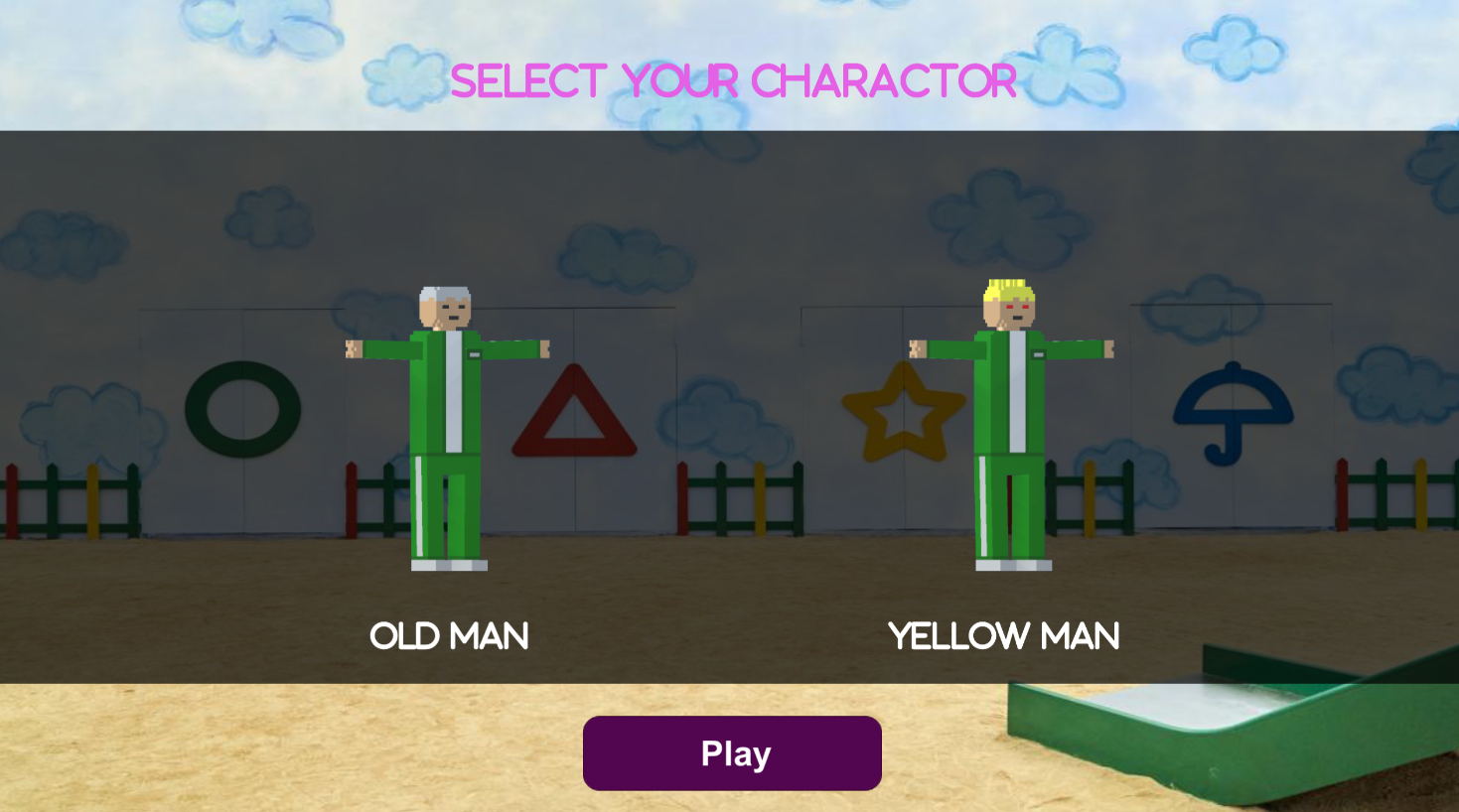
캐릭터를 선택하고 닉네임을 설정하고나면, 아래와 같이 플레이 화면으로 넘어가는것을 확인할수있다.
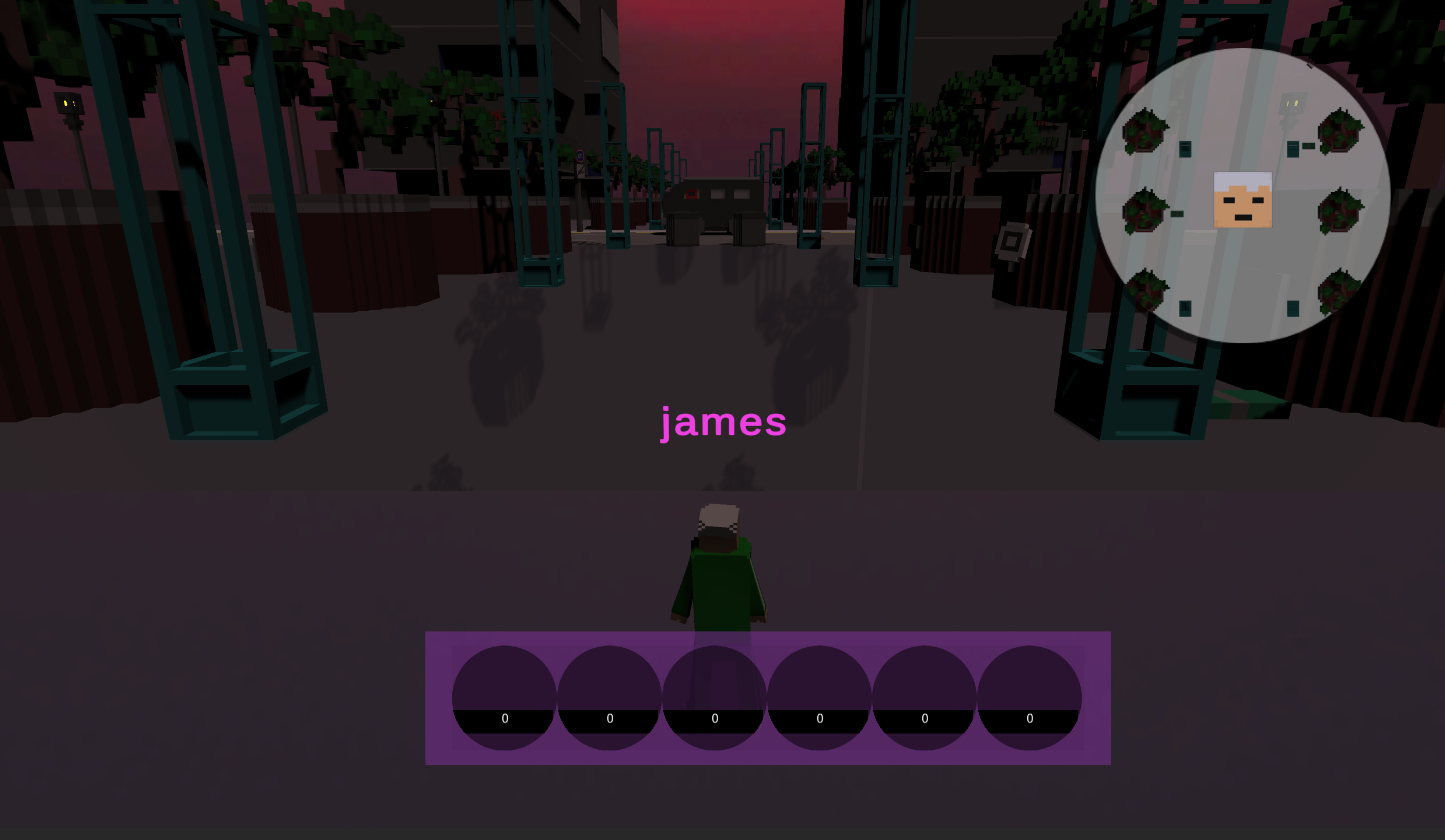
이후 아래와 같이 아이템을 습득하게 되면, 해당 순서에 따라 인벤토리에 저장되어 사용할수 있도록 구현하였다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Inventory : MonoBehaviour
{
private bool InvenOpen = false;
public GameObject InvenWindow;
public GameObject SlotParent;
private Inven_slot[] slotList;
// Start is called before the first frame update
void Start()
{
slotList = SlotParent.GetComponentsInChildren<Inven_slot>();
}
// Update is called once per frame
void Update()
{
OpenInven();
}
private void OpenInven()
{
if (Input.GetKeyDown(KeyCode.Tab))
{
if (InvenOpen == false)
{
InvenWindow.SetActive(true);
InvenOpen = true;
}
else
{
InvenWindow.SetActive(false);
InvenOpen = false;
}
}
}
public void GetItem(Item _item, int _count = 1)
{
for (int i = 0; i < slotList.Length; i++)
{
if (slotList[i].item != null)
{
if (slotList[i].item.itemName == _item.itemName)
{
slotList[i].SlotCount(_count);
return;
}
}
if (slotList[i].item == null)
{
Debug.Log(slotList.Length);
slotList[i].itemAdd(_item, _count);
return;
}
}
}
}
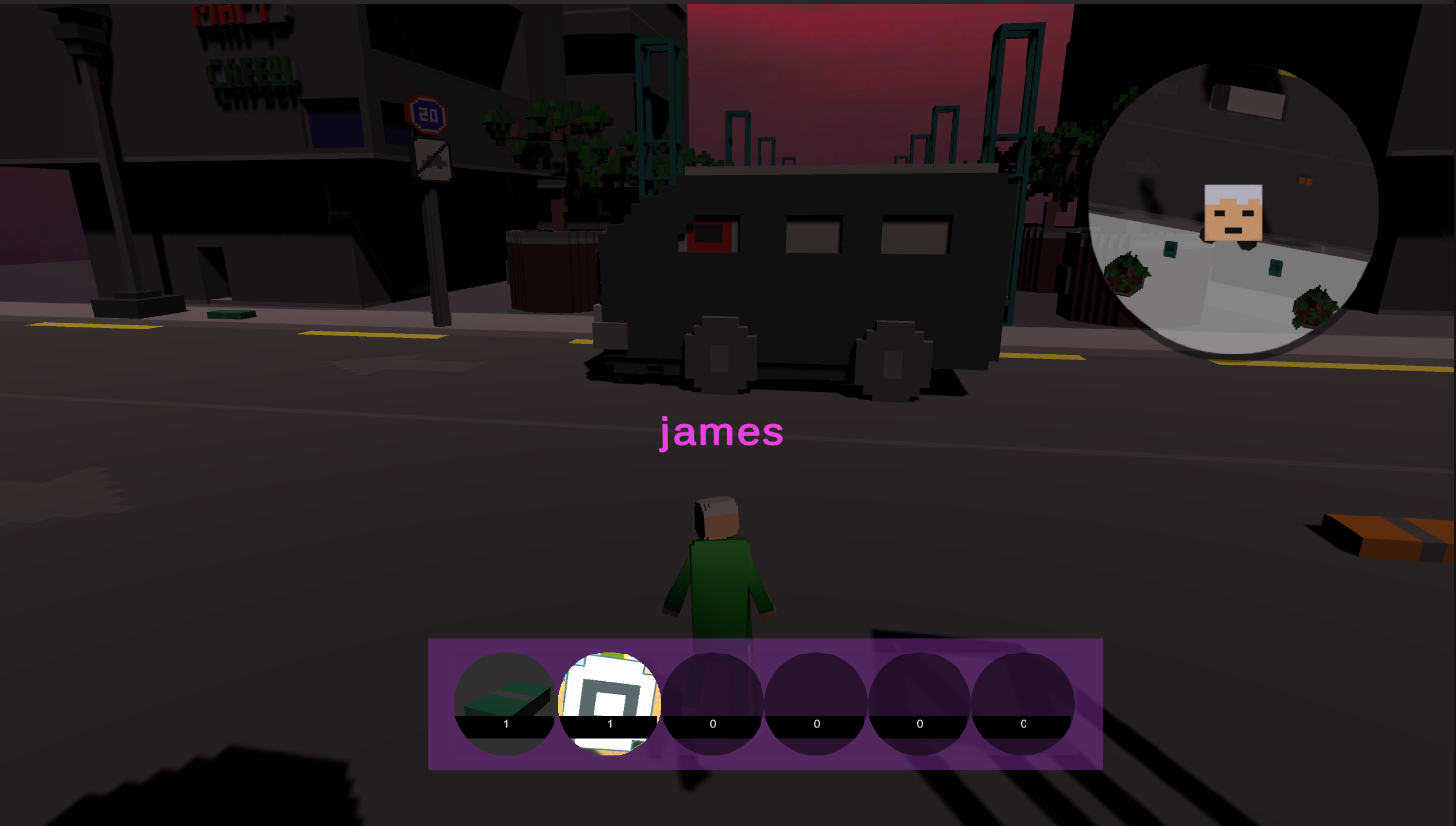
모든 아이템 습득 및 준비가 되어 다음 Scene으로 넘어간다면 아래와 같이 적들이 플레이어를 추적해온다. 이때는 미리 이동가능한 동선을 Bake 해준대로 추적을 수행하게되며, 이는 유니티 내의 AI 기능을 적용시켜, 적이 벽등에 비벼져 공중에 뜨거나, 바닥으로 꺼지는 일이 없도록 구현하였다.
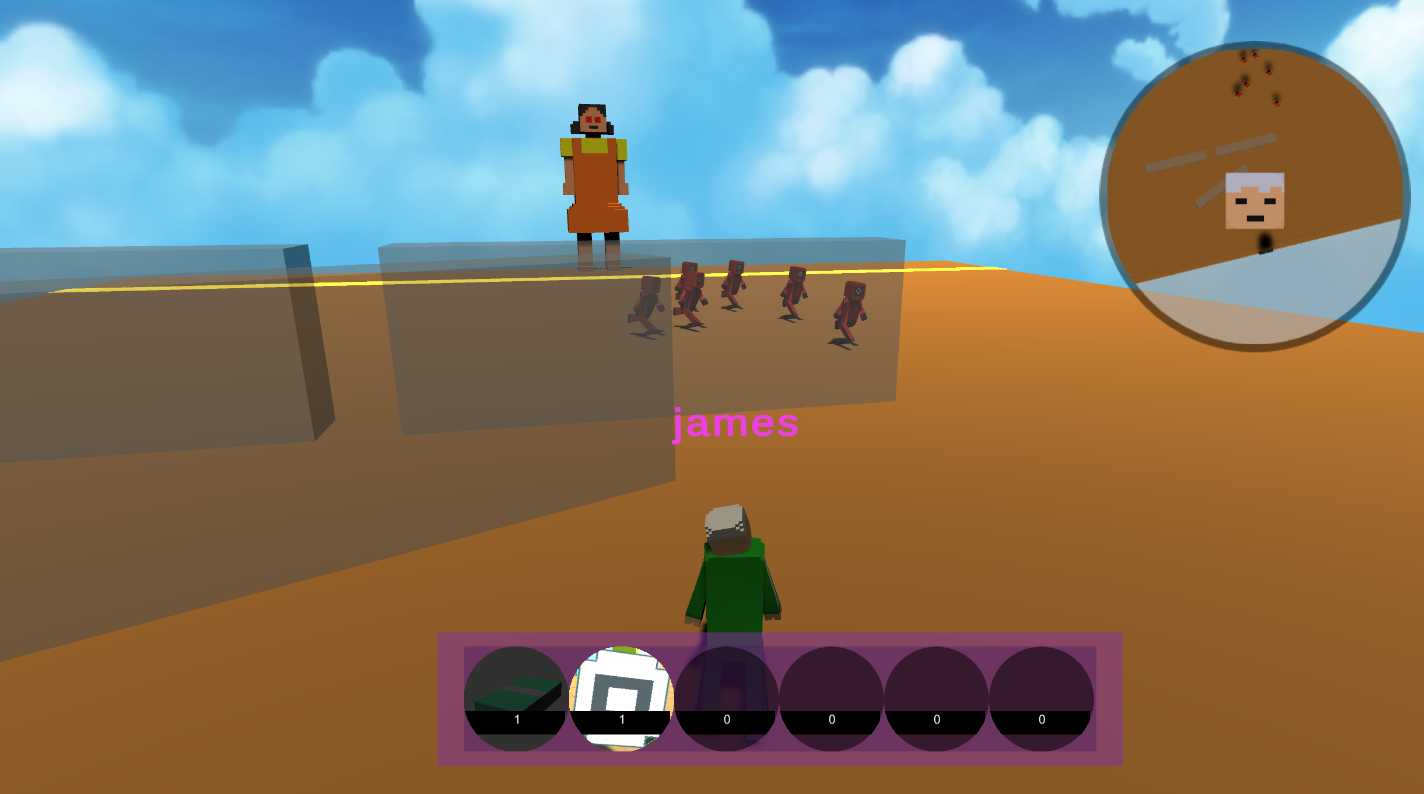
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
using UnityEngine.UI;
public class NpcMove2 : MonoBehaviour
{
public Transform player;
NavMeshAgent npc;
public Animator anim;
enum State
{
Idle,
Run
}
//상태 처리
State state;
// Start is called before the first frame update
void Start()
{
state = State.Idle;
npc = GetComponent<NavMeshAgent>();
player = GameObject.FindWithTag("Player").transform;
npc.destination = player.transform.position;
}
// Update is called once per frame
void Update()
{
if(state == State.Idle)
{
UpdateIdle();
}
else if(state == State.Run)
{
UpdateRun();
}
}
private void UpdateRun()
{
//남은 거리가 2미터라면 run 중지
float distance = Vector3.Distance(transform.position, player.transform.position);
if(distance <= 2)
{
state = State.Idle;
anim.SetTrigger("Idle");
}
npc.speed = 3.5f;
npc.destination = player.transform.position;
}
private void UpdateIdle()
{
npc.speed = 0;
//생성될때 플레이어의 위치를 찾는다.
player = GameObject.FindWithTag("Player").transform;
// 플레이어를 찾으면 Run상태로 전이
if(player != null)
{
state = State.Run;
//이렇게 state 값을 바꿨다고 animation까지 바뀔까? no! 동기화를 해주어야한다.
anim.SetTrigger("Run");
}
}
}
그 외, 습득한 아이템의 기능으로는 돈을 뿌리게 되면 적이 돈을 우선적으로 추적후, 돈을 습득하여 맵에 존재하지않게 되면 다시 플레이어를 추적하는 기능 , 가면을 장착하면 적과 동일하게 변하여 적이 플레이어를 인식하지 못하는 기능이 존재한다.
MagicalVoxel을 이용하여 프로젝트에 필요한 다양한 오브젝트들을 직접 구현하고 적용할수있다는점에서 큰 매력이 있었던것 같다. 꼭 Voxel 테마의 프로젝트가 아니더라도, 프로젝트 개발을 하는 과정에서 종종 쓰일것 같은 프로그램을 배운것 같아 뿌듯했고, 로우폴리 테마의 프로젝트를 구상중인데, 정말 많은 도움이 될것같다.
나아가 다음에는 블랜더나 마야와 같은 하이폴리 프로젝트에서 쓰일수있는 다양한 프로그램도 학습하고 싶은 의지가 생겼다 .
유데미코리아 바로가기 : https://bit.ly/3b8JGeD
Udemy Korea - 실용적인 온라인 강의, 글로벌 전문가에게 배워보세요. | Udemy Korea
유데미코리아 AI, 파이썬, 리엑트, 자바, 노션, 디자인, UI, UIX, 기획 등 전문가의 온라인 강의를 제공하고 있습니다.
www.udemykorea.com
본 포스팅은 유데미-웅진씽크빅 취업 부트캠프 유니티 1기 과정 후기로 작성되었습니다.
#유데미, #유데미부트캠프, #취업부트캠프, #개발자부트캠프, #IT부트캠프, #부트캠프후기
'STARTERS' 카테고리의 다른 글
유데미 스타터스 유니티 1기 취업 부트캠프 - 15주차 학습 일지 (0) | 2022.10.03 |
---|---|
유데미 스타터스 유니티 1기 취업 부트캠프 - 14주차 학습 일지 (0) | 2022.09.25 |
Starter Asset 스터디 정리 포스팅 [ 전처리기 지시어 (Preprocessor Directive) ] (0) | 2022.09.16 |
유데미 스타터스 유니티 1기 취업 부트캠프 - 12주차 학습 일지 (0) | 2022.09.11 |
유데미 스타터스 유니티 1기 취업 부트캠프 - 11주차 학습 일지 (0) | 2022.09.04 |